Every developer remembers their first significant project that initial leap from tutorial examples to building something real. For me, it was a Canteen Management System developed during my diploma studies. What started as a simple class project evolved into a journey that would fundamentally shape my understanding of web development.
Introduction
Every developer remembers their first significant project that initial leap from tutorial examples to building something real. For me, it was a Canteen Management System developed during my diploma studies. What started as a simple class project evolved into a journey that would fundamentally shape my understanding of web development.
Back during my diploma studies, I embarked on my web development journey by building this system alongside my friend. For me personally, this project wasn't just about creating a web application, it was my introduction to software development practices. Under his guidance, I learned crucial skills like code structuring, Git version control, and collaborative development that would shape my future career. Very appreciate that he took the time to teach me a lot these skillsets.
Five years have passed since we completed this diploma project, and while the technology landscape has evolved significantly, the fundamental lessons and problem-solving approaches remain relevant. Looking back, this project represented more than just a academic requirement, it was a hands-on crash course in software development that taught me the importance of clean code architecture and team collaboration.
Technical Stack & Tools
- Backend: Java with EntityManager
- Frontend: Java Servlet & JSP
- Database: JDBC SQL
- UI Framework: Bootstrap 4, jQuery
- Version Control: Git & GitHub
Looking at this stack in 2024, while some might consider it "legacy" but it taught us crucial lessons about:
- MVC architecture patterns
- Frontend-backend communication
- Session management and security
- Database design and optimization
- Collaborative development workflows
The Assignments
Project Overview
Our diploma project challenged us to revolutionize campus dining through technology. This system was conceived as a comprehensive web application to streamline food ordering and canteen management at our university. While the core requirement was to demonstrate proficiency in Java and SQL, we pushed beyond the basics to create a solution that addressed real student needs.
System Requirements
The system was designed around two key user groups, students and canteen staff, with distinct functionalities:
Student Features
- Digital pre-ordering system with credit-based payments
- Meal subscription and package booking
- Digital coupon redemption system
- Real-time account balance management
Staff Features
- Order management dashboard
- Digital credit top-up processing
- Inventory and stock management
- Comprehensive reporting system
- Daily sales analytics
- Inventory tracking
- Customer behavior insights
Brand Identity Journey
I tried designing BRICKS visual identity myself. However, I soon learned it's better to collaborate with experts. Working with my brother, who has graphic design experience, we developed two brand identities.
- Student Portal Theme:
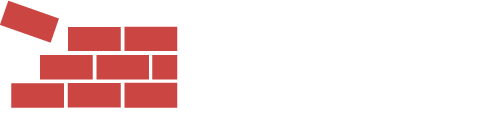
- Staff Dashboard Theme:
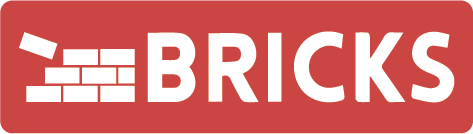
The System Design & Architecture
Our data architecture was designed to handle complex relationships between users, orders, inventory, and meal plans efficiently.
The ERD above illustrates how we structured our database to support key features like order management, inventory tracking, and subscription handling. This design enabled us to maintain data integrity while ensuring scalable performance.
User Interface Walkthrough
Student Portal Features
The student interface was designed with simplicity and efficiency in mind, offering a seamless ordering experience:
- Food Ordering Interface
Students can choose between individual items or meal packages, with an intuitive selection process that mimics popular food delivery apps.
- Category Selection
We implemented a visual category system with custom cover image for each stall type. Yeah you might be wondering why there's a category with a "Anime Girl" holding a juice. Well, thats what it means...
- Menu Exploration
The food listing page presents available items with clear pricing and descriptions. The Hakka Ramen proved to be one of our most popular items!
- Digital Coupon System
One of our innovative features was the digital coupon system that:
- Generated unique barcodes for each order
- Provided calendar view for tracking redemptions
Downside is that student still have save and crop-out the coupons themself, or to print out the physical copy of the coupons.
Staff Management Portal
The staff interface focused on operational efficiency and management capabilities:
- Order Redemption System
Staff could quickly validate and process student orders through:
- Barcode scanning
- Real-time coupon validation
- Automated status updates
- Operations Dashboard
The dashboard provided:
- Daily order summaries
- Ingredient preparation lists
- Real-time kitchen management tools
- Menu Management
Staff could easily:
- Update menu items
- Manage ingredient requirements
- Adjust pricing and availability
- Package Management
The package management system enabled:
- Weekly meal planning
- Subscription management
- Dynamic package customization
- Analytics Dashboard
Comprehensive reporting features included:
- Revenue tracking
- Order volume analysis
- Monthly report generation
- Exportable data formats
TIP
In addition, there are still more screenshots and technical details to cover. If you're interested, you can always check out our complete project documentation.
Code Review?
Revisiting code from five years ago offers a unique perspective on both technical growth and fundamental programming principles. While frameworks and tools have evolved, the core concepts remain remarkably relevant.
Modular Design Patterns Then and Now
Consider this snippet from our JSP
implementation:
// web/staff/package/addPackage.jsp
<%@page import="entity.Meal" %>
<%@page import="service.MealService" %>
<%@page import="java.util.List"%>
<%
MealService mealService = new MealService();
List<Meal> MealList = mealService.findMealByAvailability(true);
%>
<!doctype html>
<html lang="en">
<head>
<%@include file="../layout/meta.jsp" %>
<%@include file="../layout/css.jsp" %>
<title>Staff | Manage Packages</title>
<!-- ... -->
What's fascinating is how this code demonstrates timeless principles that remain relevant in modern development:
- Modularity: Using
JSP
includes for layouts (similar to modern component systems) - Service Layer Pattern: Separating business logic with
MealService
- Object-Oriented Design: Leveraging entity classes like
Meal
Meanwhile...
Looking at my commit history brings both nostalgia and learning opportunities
Yeah, I missed those days were fun and exciting when we able to solve the bugs on our own. While these commit messages showcase the excitement of problem-solving they also highlight how development practices mature over time. Today's best practices for commit messages emphasize:
TIP
- Clear, descriptive messages
- Consistent formatting
- Separation of concerns
Reflections & Takeaways
Key lessons from this project that still hold true:
- Fundamental Principles Endure:
- SOLID principles
- Object-Oriented Programming
- Modular design patterns
- Technology Evolution:
- While syntax evolves (
JSP
→React
/Vue
) - Core concepts remain unchanged
- Focus on fundamentals pays long-term dividends
- While syntax evolves (
- Development Process Growth
- From exciting commits to structured change management
- Documentation importance
- Code organization and maintainability
For those interested in the project repository, you can find it on our GitHub!
Thank you for joining me on this nostalgic journey through my first major project. While our implementation might seem dated now, the learning experience and foundational knowledge gained continue to influence my development approach today.